HWJ12_Clock
In an applet named HWJ12_Clock, create the seconds hand for a clock.
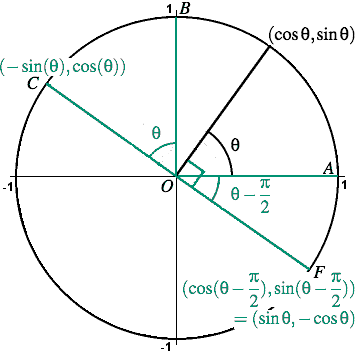
Sine and cosine are part of the Math
class. To use sin its:
Math.sin(someRadianNumber); //this would give us the
sin value of someRadianNumber
Sin and cosine take in a radian measurement and return the value.
If we wanted to find the sin of a degree like 45 degrees we would
say:
Math.sin(Math.toRadians(45)); //this would return
the sin value of 45 degrees
All points on the unit circle are (Cos, Sin)
All points on any circle are (radius*Cos, radius*Sin)
Start with the code from timing.
Heres code from class:
int radius=100; //the radius of my circle
int angle=140;
int x2=250+(int)(radius*Math.cos(Math.toRadians(angle)));
int y2=250-(int)(radius*Math.sin(Math.toRadians(angle)));
g.drawLine(250,250,x2,y2);
g.drawString("x2 is:"+x2,100,100);
g.drawString("y2 is:"+y2,150,100);
|